How to retrieve the base64 encoding for any image in Go
The base64 encoding of an image is useful in several contexts. It commonly used when there is a need to store or transfer images over media that are designed to deal with textual data. This post will describe how to retrieve the base64 representation of any image using the Go standard library
Converting an image to its base64 representation is easy to do in Go. All you
need to do is read the file as a byte slice and encode it using the
encoding/base64
package.
Local images
First, let’s handle the situation where the image file resides locally on the filesystem.
package main
import (
"encoding/base64"
"fmt"
"io/ioutil"
"log"
"net/http"
)
func toBase64(b []byte) string {
return base64.StdEncoding.EncodeToString(b)
}
func main() {
// Read the entire file into a byte slice
bytes, err := ioutil.ReadFile("./file.png")
if err != nil {
log.Fatal(err)
}
var base64Encoding string
// Determine the content type of the image file
mimeType := http.DetectContentType(bytes)
// Prepend the appropriate URI scheme header depending
// on the MIME type
switch mimeType {
case "image/jpeg":
base64Encoding += "data:image/jpeg;base64,"
case "image/png":
base64Encoding += "data:image/png;base64,"
}
// Append the base64 encoded output
base64Encoding += toBase64(bytes)
// Print the full base64 representation of the image
fmt.Println(base64Encoding)
}
The first step is to read the image file into a slice of bytes through
ioutil.ReadFile()
. Next, the http.DetectContentType()
is used to determine
the MIME type of the
file so that the appropriate data URI scheme header is prepended to
the base64 encoded output. Afterwards, the toBase64()
function is invoked
which uses the base64.StdEncoding.EncodeToString()
method to retrieve the
base64 encoding of the image. The result of this function call is appended to
the URI scheme header.
Once you run this code, it will print the base64 encoding of the image to the
standard output provided file.png
exists on the filesystem.
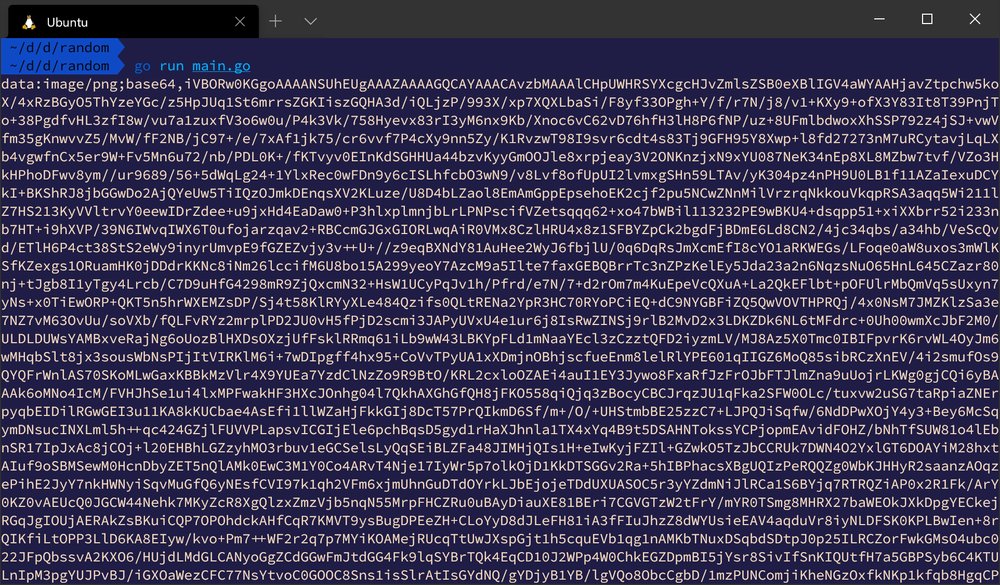
You can copy the base64 string and paste it directly to your browser’s address bar. It should display the entire image as shown below.
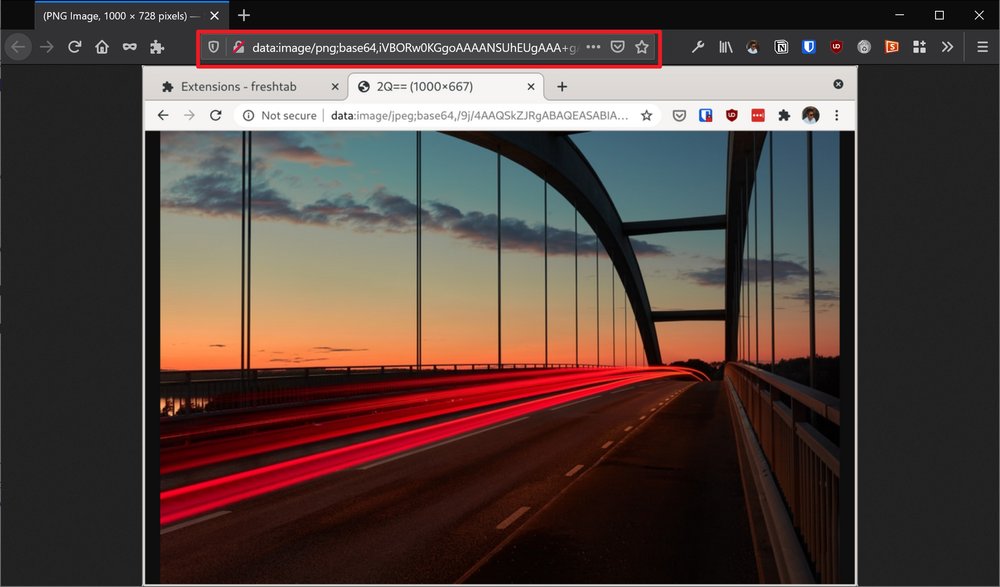
Remote images
Converting an image that resides at a URL to its base64 encoding isn’t much different from what we’ve already seen above. All you need to do is make a GET reequest for the image and then read the response body into a slice of bytes. Afterwards, the rest of the process is identical to when working with local images.
package main
import (
"encoding/base64"
"fmt"
"io/ioutil"
"log"
"net/http"
)
func toBase64(b []byte) string {
return base64.StdEncoding.EncodeToString(b)
}
func main() {
resp, err := http.Get("https://freshman.tech/images/dp-illustration.png")
if err != nil {
log.Fatal(err)
}
defer resp.Body.Close()
bytes, err := ioutil.ReadAll(resp.Body)
if err != nil {
log.Fatal(err)
}
var base64Encoding string
mimeType := http.DetectContentType(bytes)
switch mimeType {
case "image/jpeg":
base64Encoding += "data:image/jpeg;base64,"
case "image/png":
base64Encoding += "data:image/png;base64,"
}
base64Encoding += toBase64(bytes)
fmt.Println(base64Encoding)
}
Thanks for reading, and happy coding!